C#中随机数出现完全重复的情况
using System;
using System.Collections.Generic;
using System.Text;
namespace ContainerTests
{
class Part2
{
// Make A Dict By [stringKey:intValue]
static Dictionary<string, int> MakeRandomDict()
{
Random random = new Random();
Dictionary<string, int> dict = new Dictionary<string, int>();
string aToZ = "abcdefghijklmnopqrstuvwxyz";
foreach (char key in aToZ){dict.Add(key.ToString(), random.Next(1, 11));}
return dict;
}
// Just Loop Print Key && Value
static void PrintDict(Dictionary<string, int> dict)
{
foreach (KeyValuePair<string, int> kV in dict)
{
Console.WriteLine(kV.Key + ":" + kV.Value);
}
}
// Remove Even Value Item
static void RemoveAnEvenValueFromDict(Dictionary<string, int> dict)
{
// This List Save Remove Keys...
List<string> removeKeys = new List<string>();
foreach (string key in dict.Keys)
{
if (dict[key] % 2 == 0)
{
removeKeys.Add(key);
}
}
foreach (string removeKey in removeKeys)
{
dict.Remove(removeKey);
}
}
// Do Merge Dict
private static Dictionary<string, int> LeftJoinMergeDict(Dictionary<string, int> dictA, Dictionary<string, int> dictB)
{
foreach (string key in dictB.Keys)
{
// Sure , If Not Exsits , Added it
if (!dictA.ContainsKey(key))
{
dictA.Add(key, dictB[key]);
}
}
return dictA;
}
static void Main(string[] args)
{
/* (5分) 1、对字典dictA,删除所有值为偶数的键值对。
2、再次创建同样的字典dictB,也同样删除值为偶数的键。
3、然后将这两个字典合并成一个新字典。Key相同的部分,以dictA的值为准*/
Dictionary<string, int> dictA = MakeRandomDict();
// Do Remove
RemoveAnEvenValueFromDict(dictA);
Console.WriteLine($"-------------------This is A Dict After Result Size {dictA.Count} -----------------------");
// Print After Result
PrintDict(dictA);
Dictionary<string, int> dictB = MakeRandomDict();
// Do Remove
RemoveAnEvenValueFromDict(dictB);
Console.WriteLine($"-------------------This is B Dict After Result Size {dictB.Count} -----------------------");
// Print After Result
PrintDict(dictB);
Dictionary<string, int> dictC = LeftJoinMergeDict(dictA, dictB);
Console.WriteLine($"-------------------This is Merge Dict Size {dictC.Count} -----------------------");
// Print After Result
PrintDict(dictC);
Console.ReadLine();
}
}
}
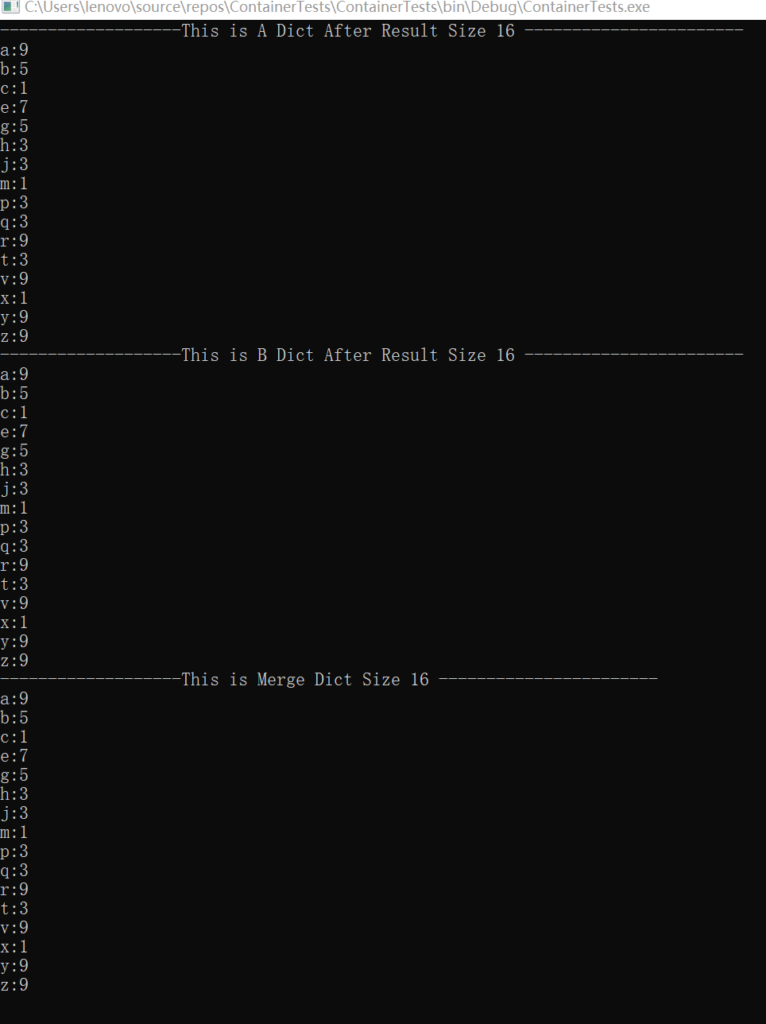
如图👆,居然打印出了完全一样的值,而且多次尝试重新运行,有时候是不同的,有时候就是这种情况。
改善的方法:
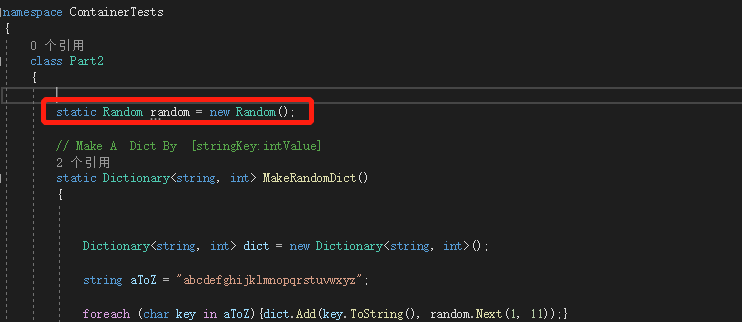
经过测试发现不会出现完全相同的随机值。
猜测 random 方法的Next计算和系统时间戳有关系。
而代码的运行可能效率非常高,导致上下两次调用时间无线接近,这样一来 也许random的seed是一样了。
Comments | NOTHING