Eclipse Ditto 是数字孪生模式提供对物联网设备的访问。
为了给不同的设备提供结构化的 API,Ditto 定义了一个基于 JSON 的轻量级 JSON 模型。
可能类似于以下示例:
{
"thingId": "the.namespace:the-thing-id",
"policyId": "the.namespace:the-policy-id",
"attributes": {
"location": "kitchen"
},
"features": {
"transmission": {
"properties": {
"cur_speed": 90
}
}
}
}
IoT 中的设备,可能是各式各样各地设备,【可能不会按照Ditto 要求的结构和协议将其数据发送到云端。】
他们不需要知道像 Ditto 这样在云中运行的东西会将他们做成数字孪生体。
因此,例如设备传过来的数据可能如下所示:
{
"AHU-ENA":"123",
"C-1":"456",
"C-2":"1234",
"EAD-C":"241",
"EF-AM":"123",
"EF-C":"321",
"EF-F":"321",
"EF-S":"321",
"EFVSD-C":"1234",
"EFVSD-FB":"523123",
"FA-H":"2135",
"FA-T":"123125",
"FFILT-S":"521",
"HA-H":"123",
"HA-T":"4321",
"HUM-C":"567",
"JDGL-S":"743",
"LOWT-A":"345",
"OAD-C":"643734",
"OAD-MIN":"74436",
"RA-H":"",
"RA-HSP":"",
"RA-T":"",
"RA-TSP":"",
"RAD-C":"",
"RM-CO2":"",
"RM-CO2SP":"",
"RM-H":"",
"RM-T":"",
"SA-SP":"",
"SA-SPSP":"",
"SA-T":"",
"SF-AM":"",
"SF-C":"",
"SF-F":"",
"SF-S":"",
"SFVSD-C":"",
"SFVSD-FB":"",
"VLV-C":"",
"VLV-MIN":"",
"VSD-MIN":"",
"WS-EX":""
}
在受限设备或物联网协议的情况下,甚至二进制也可能很常见。
0x08BD (hex representation)
So , Ditto 提供了内置的出入站映射来帮助你解决问题
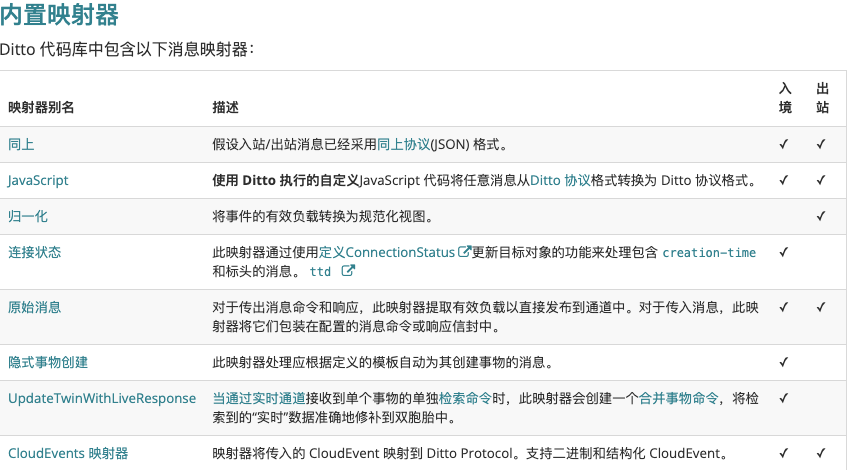
下文主要讲一下【JavaScript 映射器】
只要任何入站消息尚未在Ditto Protocol中,就可以使用此映射器。通过使用内置的JavaScript 映射引擎(基于 Rhino),可以执行自定义定义的 JavaScript 脚本,这些脚本负责 从任意消费的数据创建Ditto Protocol JSON消息。
为了将Ditto协议 JSON 消息(例如事件或响应)转换为任意其他格式,出站消息也是可能的。
配置选项
incomingScript
(必需):传入消息的映射脚本outgoingScript
(必需):传出消息的映射脚本loadBytebufferJS
(optional, default:"false"
): 是否加载ByteBufferJS库loadLongJS
(optional, default:"false"
): 是否加载LongJS库
JavaScript 映射引擎
Ditto 利用适用于 Java 的Rhino JavaScript 引擎来评估 JavaScript 以申请映射有效数据。
使用 Rhino 而不是 Nashorn,这是随 Java 一起提供的较新的 JavaScript 引擎,它的好处是可以更好地应用沙盒。
需要对不同的有效负载脚本进行沙盒处理,因为 Ditto 在作为云服务运行,其中同时为不同的租户管理到不同端点的多个连接。这需要隔离每个单独的脚本,以避免干扰其他脚本,并保护执行脚本的 JVM 免受有害代码的执行。
约束条件
Rhino 不完全支持 EcmaScript 6。在映射函数中使用它们之前,请检查支持哪些语言结构。请参阅http://mozilla.github.io/rhino/compat/engines.html。
Ditto 当前包括 Rhino 版本1.7.14
并VERSION_ES6
启用了标志。
出于沙盒/安全原因,以下限制适用:
- 无法访问 Java 包和类
- 无法使用
exit
,quit
,等print
- 无法访问文件
- 无法进行远程调用(例如对外国网络服务器)
- 编写无限循环将终止脚本
- 编程递归将终止脚本
- 脚本的文件大小是有限的
- 不能加载外部 JS 库(除非它们符合文件大小限制并包含在映射脚本中)
JavaScript 示例
文本负载示例
假设您的设备通过Eclipse Hono 的MQTT 适配器将遥测数据发送到云中。并且,您设备的示例有效载荷是:
{
"temp": "23.42 °C",
"hum": 78,
"pres": {
"value": 760,
"unit": "mmHg"
}
}
我们希望将包含所有 3 个值更新的此设备的单个消息映射到以下结构中的事物:
{
"thingId": "the.namespace:the-thing-id",
"policyId": "the.namespace:the-policy-id",
"features": {
"temperature": {
"properties": {
"value": 23.42
}
},
"pressure": {
"properties": {
"value": 760
}
},
"humidity": {
"properties": {
"value": 78
}
}
}
}
因此,我们定义如下incoming
映射函数:
function mapToDittoProtocolMsg(
headers,
textPayload,
bytePayload,
contentType
) {
if (contentType !== 'application/json') {
return null; // only handle messages with content-type application/json
}
let jsonData = JSON.parse(textPayload);
let value = {
temperature: {
properties: {
value: jsonData.temp.split(" ")[0] // omit the unit
}
},
pressure: {
properties: {
value: jsonData.pres.value
}
},
humidity: {
properties: {
value: jsonData.hum
}
}
};
return Ditto.buildDittoProtocolMsg(
'org.eclipse.ditto', // 请替换它为你的Namespace
headers["device_id"].split(":")[1], // Eclipse Hono sets the authenticated device_id as AMQP 1.0 header
'things', // we deal with a Thing
'twin', // we want to update the twin
'commands', // we want to create a command to update a twin
'modify', // modify the twin
'/features', // modify all features at once
headers, // pass through the headers from AMQP 1.0
value
);
}
当您的设备现在通过 Eclipse Hono 的 MQTT 适配器发送其有效数据时:
mosquitto_pub -u 'sensor1@DEFAULT_TENANT' -P hono-secret -t telemetry -m '{"temp": "23.42 °C","hum": 78,"pres": {"value": 760,"unit": "mmHg"}}'
映射就工作了!
原文文档地址: https://www.eclipse.org/ditto/connectivity-mapping.html#bytes-payload-example
Comments | NOTHING